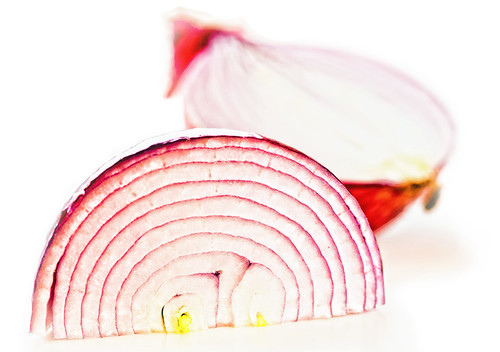
For the last couple weeks we’ve been going through an introduction to how websites work by diving in head first (no pun intended) and looking at working code. While this does help you understand how your website works and how to customize the head section for your own website, there’s also a lot it doesn’t do. It doesn’t give you the basic rules you need for writing HTML code. It doesn’t give you the power to develop an entire website, or to read and understand any and all HTML code, whether it be in the head or elsewhere.
So I’m going to lay down some rules, and a scattering of tips about HTML.
What, Were You Born In A Barn?
When you were growing up, your parents probably taught you not to leave the door wide open after you go through. And if you forgot, there was probably someone who would ask, “What, were you born in a barn?” Now, I have nothing against people born in barns. And sometimes, it makes sense to leave the door open. In HTML, however, it is incredibly important to always remember to close what you’ve opened.
Every time you open a tag, you must remember to later close it. Every. Single. Time. This is of utmost importance, because if you don’t, you can completely screw up the layout of your entire webpage. And then you’ll be running around for half an hour, pulling your hair out and trying to figure out why your sidebar won’t go where it’s supposed to, until you find that the cause was simply a missing closing tag.
ahem Not that I speak from experience or anything.
“But… but… but… there are exceptions to that rule, right? What about that <br />
tag, or the <img />
tags? You only ever see them by themselves… they don’t need closing tags, right?”
Nope. No exceptions. Every single tag must be closed. For every <p>
there must be a </p>
. (Remember two weeks ago, I told you that the backslash at the beginning means it’s a closing tag, whereas if there’s no backslash, then it’s the opening tag.)
However. Take a look at the <br />
tag. There’s no backslash at the beginning. So that means it’s an opening tag. But… there is a backslash at the end. So wait… does that also mean it’s a closing tag?
Yes. Yes, it does. Looking at it that way, it makes sense for some tags to be both opening and closing tags at the same time – such as the <br />
tag, which just inserts a line break (a carriage return), or the <img />
tag, which inserts an image into your page.
So what have we learned? You always have to close your tags – whether it be in the opening tag itself (<br />
) or in a separate closing tag (<p></p>
).
No, I’m Not Crying, Just Cutting Onions
Go ahead, slice open an onion. (Try not to cry.) What do you see? A bunch of rings. But not a jumble of rings, overlapping one another haphazardly like a messy drawer of jewelry. No, you see organized circles, neatly nested one within another. They never overlap like the orbits of Neptune and Pluto (which is a planet, goshdarn it!).
Your code should be an onion. (Eh? But I don’t see rings in my code…) Every element should be like a ring – it should enclose or be enclosed by other elements, but never overlap. That may sound confusing, but bear with me–it’ll make sense once you see the actual code.
This is a bare bones example of what the body of your typical webpage might look like:
<body> <div id="header"> <a href=""> <img src=""> </a> <div id="navigation"> <ul> <li> <a href="">Home</a> </li> <li> <a href="">About</a> </li> </ul> </div> </div> <div id="content"> <div id="main"> (main content stuff) </div> <div id="sidebar"> (sidebar stuff) </div> </div> <div id="footer"> (footer stuff) </div> </body>
You’ll see that your code is LIFO – Last In, First Out. What that means is the last tag you opened is the first tag you’ll close. Always, always, always. Similarly, the first tag you open will be the last one you close.
Let’s go step by step through that example. First, you see the <body>
element. That’s the very first tag you open with, and the very last tag you close. Since it encapsulates everything, it’s like the outermost skin of an onion.
Then you’ve got the subsections inside – the ‘header’ div, the ‘content’ div, and the ‘footer’ div. You know how some onions have more than one ‘center’, like that one in the picture up top? Almost like there are two baby onions growing inside one big one. That’s what this is like. You’ve got three littler onions (your subsections) inside the big one (the body element). And each of those littler onions can contain even smaller ones.
To help you see the ‘rings’, we indent every level. <body>
is on the outside, so it’s indented the least. Then the ‘header’, ‘content’, and ‘footer’ divs are on the next level, because they’re inside the <body>
element. By doing this, it’s easier to see that your rings aren’t overlapping, and that every element closes.
So what happens if you don’t nest your elements properly, if your rings overlap? Well, take a look:
<div id="header"> <a href=""> <img src=""> <div id="navigation"> </a> <a href=""></a> <ul> <a href=""> <li> </a> <a href=""></a> <a href="">Home</a> </li> <li> <a href="">About</a> </li> </ul> </div> </div> <div id="content"> <div id="main"> <div id="sidebar"> </div> </div> </div> <div id="footer"> </div>
Yeah… it’s a complete mess. Not only is it difficult for you to read, but your browser isn’t going to have the faintest idea what to do with it. So remember: Nest. Like the rings of an onion. Always. It is of utmost importance.
Please, No Yelling!
(X)HTML tags are always lowercase. Always. Always.
But why? Well, for one thing, us coders don’t like to feel like we’re being yelled at. I mean sure, you might yell, scream, curse out your code, and threaten to toss your computer out the window, but your coder is only trying to help. Be nice.
Okay, maybe I’m being a little goofy. But think of your code as a blog post. If you saw a post that was entirely written in uppercase letters, you’d be gone in a flash, right? Why? Because it’s difficult to read.
Same goes for your code – you want it to be easy to read. I promise you – if you ever go back and read your own code, or somebody else has to use your code, and it’s all caps, or a mixture of upper- and lower-case letters… they’re going to be cursing you. I guarantee it.
Using the same analogy, you also want your code to be properly formatted. This means properly nesting your elements, like this:
<div id="header"></div> <div id="content"> <div id="main"></div> <div id="sidebar"></div> </div> <div id="footer"></div>
not like this:
<div id="header"></div> <div id="content"> <div id="main"></div> <div id="sidebar"></div> </div> <div id="footer"></div>
See how much more difficult it is to figure out what’s going on when it’s improperly nested? You wouldn’t want your blog post to be a big mass of text without paragraphs and headers separating your thoughts. Don’t do that with your code either.
Grab Your Top Hat And Your Ball Gown…
Next week we’re getting classy.
Erm, not that SushiCodes isn’t a classy establishment already. What I mean is, next week we’re looking at the ‘class’ and ‘id’ attributes of your elements, and talking about the many, many wonderful things that can be done with them.
But you can still wear heels or a tie if you want.