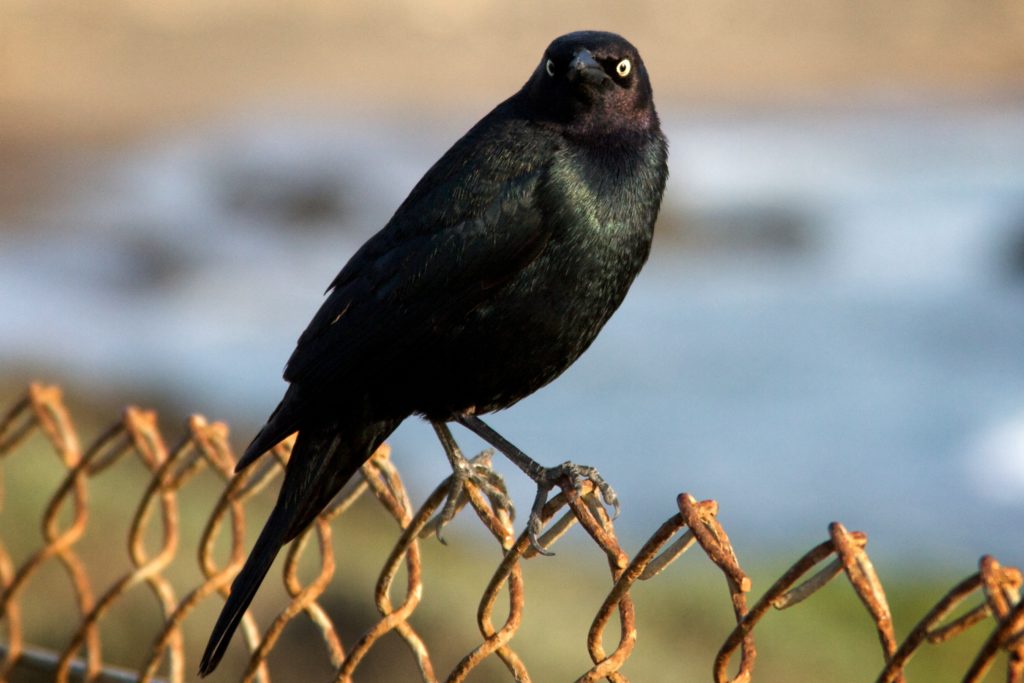
The sidebar is all sorts of useful. There’s no limit on the number of different sorts of things that could go in a sidebar. Your RSS button? Definitely. Ads? For sure! A video? Heck, why not?
You can even have multiple sidebars… one on each side of your content, or two right next to each other, on the same side of your content. Cool, isn’t it?
Some things are pretty easy. Your RSS button is likely an image, a link, and maybe some text or an email sign-up form. Ads aren’t much different. For videos, you just have to insert the code YouTube (or whatever video site you’re using) gives you for embedding. With basic knowledge about HTML, you can certainly figure out how to do these things.
But what if you want to do something more involved… like list your most recent posts in your sidebar, or put WordPress widgets in your sidebar, or have multiple sidebars, or even have different sidebars on different pages of your website? That involves a little more than just knowing how to use HTML tags and nest them properly. For that, we have to get into PHP a bit.
What have you been up to lately?
Something that’s super useful to your readers (and can help your recent posts get more traffic, which is always good!) is to have a short list of your most recent posts in your sidebar. Usually this list includes links to the five most recent posts. In theory, this is pretty easy to do. You just have to call the get_posts();
PHP function (you remember what PHP is, right?), pass in the appropriate parameters, and voila, there you go!
But this ain’t no computer science class, and I’m not some snobby professor who expects you to instantaneously understand every piece of code I throw at you. Here, we’re all about understanding things… really, truly understanding them. So we’re going to go through this line by line.
First, the code:
<h2>Recent Posts</h2> <ul> <?php $recent = get_posts('posts_per_page=5') ?> <?php foreach ($recent as $post) : ?> <li> <a href="<?php echo(get_permalink($post->ID)) ?>"> <?php echo($post--->post_title) ?> </a> </li> <?php endforeach; ?> </ul>
That first line’s easy enough to understand, right? It’s just your title – “Recent Posts” that you put in a header tag. Really, you can name it whatever you’d like, but we’re going to name it “Recent Posts” for now.
Next, the opening <ul>
tag. The function we use to display the list of posts outputs an array. We want to take this array, and turn it into a list… an unordered list, so we use the <ul>
tag.
The next line isn’t HTML at all. But you recognize that style of code, right? The tags at the beginning and the end of the line tell you that it’s PHP. Then we assign whatever we get from the get_posts()
function to a variable called $recent
– you’ll see why in a moment, but first I want to explain this function to you.
The get_posts() function does exactly what it says it does. It gets your posts! But how many? And what if you want only the ones from a certain category, or ordered a specific way, or from a specific date…? That’s what parameters are for. Here, we’re just using one parameter – posts_per_page
– to tell the function that we only want to display five posts. But there are lots more possible parameters you can use… check out these two pages to see a list of all the possible parameters that can be used with this function.
Now that we’ve got the information we need and have assigned it to a variable, we need to display it. In order to do this, we need to use a loop. Since the information is outputted in an array, we need to use a foreach loop. It’s not as hard as it looks. We’re pretty much saying we want to look at each thing in the $recent
array, and we’re going to call it a $post
. Then we do the stuff inside of the foreach loop… and continue to do it to each thing in the $recent
array, until there aren’t any left.
So what is it that we’re doing inside of the foreach loop? Well, we’re displaying the recent posts, that’s all. Since we decided that this should be an unordered list (hence the <ul>
tags) then each item in the list needs to be in <li>
tags. Easy. Then we need to display the actual recent posts – each of which needs to consist of a link (because what’s the point of a list of your recent posts, if you can’t easily get to them?) and the title of the post. We start out as we would for any ordinary link – with an <a href="">
. Then we need the link itself inside those quotes.
The cool thing with these items in the $recent
array is that each item has certain other values associated with them. So you can say $post->ID
and it will give you the ID of the post, or $post->post_title
to get the title. Awesome, right? So we use $post->ID
in conjunction with the get_permalink()
function (which takes in an ID of a post and returns the link for that post) to get the link. Remember to surround that with <?php
and ?>
tags to indicate that this is PHP code, and use the echo()
function to display the output (otherwise it just sits there, and your browser doesn’t know what to do with it.)
After that, you need some text for the link, so it’s super easy to echo $post->post_title
, which – you guessed it! – gives you the title of the post. Then just close your tags, end the loop… and voila! You have a list of your most recent posts!
More sidebar magic
Recent posts are awesome, and definitely good to have in your sidebar. But there’s more to the sidebar than just its content… what if you want to use the built in WordPress sidebar widgets, or have different sidebars on different pages of your website? You can be sure there’s much more fun to be had with the sidebars… next week!