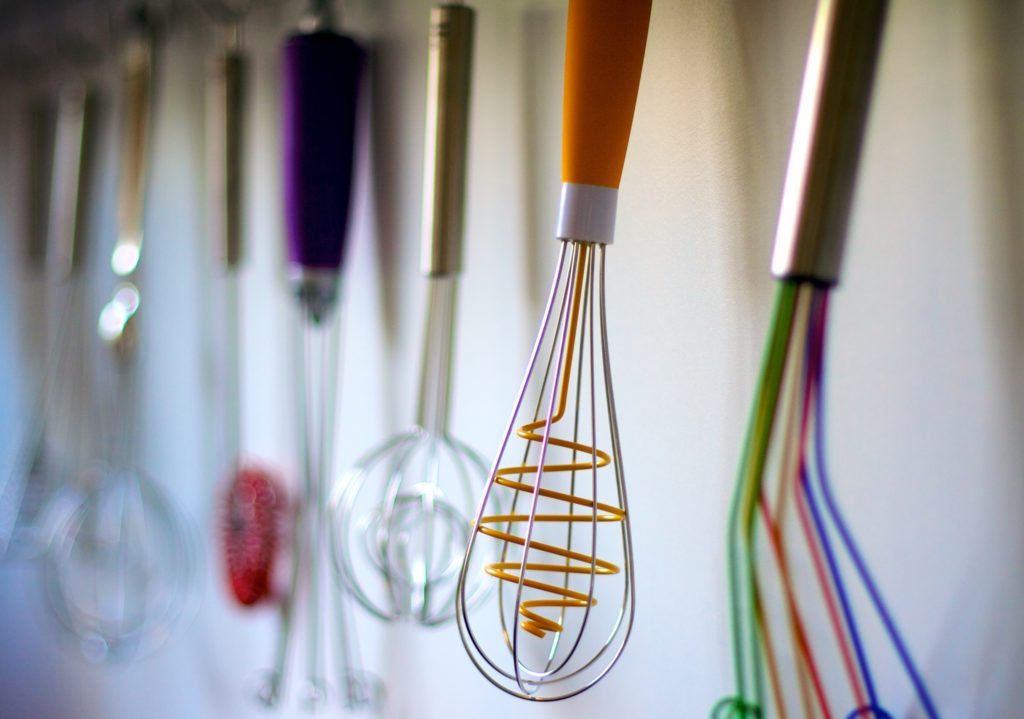
If you think back to last week’s introductory post about loops in PHP, you’ll remember I gave you some pseudo-code that described a program to run a game of hangman. And then I left you hanging.
(Yes, that pun was totally intended.)
And then we played a game of hangman in the comments. It was awesome.
But you’ll recall that I did promise to go into detail about exactly what the while, for, and for each loops are, and how they work. That’s exactly what I intend to do today. So, first things first. Take a look once more at the pseudo-code that I introduced you to last week:
choose the word to be guessed; set the hangman to empty; while (the word is incomplete and the hangman is incomplete) { tell the person the game is still going; $match = false; $guess = the persons guess; for (each letter in the word) { if (letter is the same as $guess) { $match = true; remember which position this letter goes in; } } if ($match == true) { display positions where the letter is the same as the $guess; } else { add a part to the hangman; } }
For the most part, that seems to all make sense, right? Everything is in plain English, and it tells you exactly what the code in the program is doing. But then you see the while loop, and the for loop… what does it all mean? Let’s go from the outside-in.
While
While a condition is still true, then continue looping. This is probably the easiest of the three, because you’ve seen something of a similar layout before. Remember from my article about if-statements a couple of weeks ago, that the stuff between those parentheses after ‘while’ and before ‘{‘ are the conditions. They’re the things that you test for truthiness, and if they are true, then you do the stuff that’s between the curly braces.
So here we’re saying,
while (the word is incomplete and the hangman is incomplete) { do stuff }
If the word is incomplete, and the hangman is incomplete, that means that the player has neither won nor lost the game yet, right? They still have chances to guess more letters. So that’s pretty much saying that while the game is still going, then keep doing all that stuff between the curly braces. The reason we use a while statement for this is because we don’t know how long the game will be going. If it’s a short word, you could win or lose in three tries, or it could also get up to 26 guesses.
Side note: the while loop tests the condition before it runs the code between the braces. But what if you wanted the condition to be tested after each time you run the code? Then you would use a do-while loop. Like this:
do { the stuff you want to do } while (your condition);
Pretty much the same as a normal while-loop, just… backwards.
For
For every integer starting at one number and ending at another, incrementing by a certain amount each time, loop once.
It sounds a lot more complicated than it actually is. I promise.
For-loops generally look like this:
for ($i=0; $i < 10; $i++) { do stuff }
Let’s break it down. $i=0
tells you that you have a variable $i
that starts at zero. $i<10
means you want it to continue looping so long as it is less than ten. And that last bit, $i++
, tells you that $i
should increment by one at the end of every loop. So it will run through the code inside of the loop when $i
is 0, 1, 2, 3, 4, 5, 6, 7, 8, 9… and then it will stop when $i == 10
because it is no longer less than ten. Your variable doesn’t have to be called $i
(though it quite often is). It can start at any integer and end at any integer. And while you’ll usually see the simple increment-by-one expression that we used here at the end, you can have any sort of expression at all that you want to use.
But wait… that doesn’t look like the for-loop that’s in the pseudo-code.
You’re right. So instead, look at what it would look like in real code:
for ($i=0; $i < strlen($word); $i++) {}
See? Now it looks exactly as a for-loop should. (That strlen() function simply gets the length of the string… or in our case, the length of the word that the player is supposed to be guessing, so we can loop through every letter to see if it’s a match.)
For Each
For each thing in some sort of group of things, loop once. This group of things is known as an array. “But wait,” you wonder, “what’s an array, and why on earth would I use one?” An array is nothing more than a grouping of things. Yes, I know, I just said that. But it’s no more complicated than that. Imagine you have a handful of coins. (An array of coins.) And you want to put them in a piggy bank, but it’s got a tiny slot so you can only put one in at a time. So you might do it like this:
foreach ($handfulOfCoins as $coin) { put coin in piggy bank }
$handfulOfCoins
… that’s your array. And you’re saying that each thing in this array is a $coin
, and you want to do the code inside the brackets to every single $coin
in your $handfulOfCoins
.
Now That Wasn’t So Hard, Was It?
You’re catching on. I just know you are. In fact, I have so much faith in you… next week, I’m going to show you what the real code for this hangman program looks like! And, even better, we’re going to code our very first real PHP program!! Aren’t you excited? I know I am. It’s going to be awesome.
1 Comment
Pingback: PHP: Real Code, And A Game Of Hangman – SushiCodes