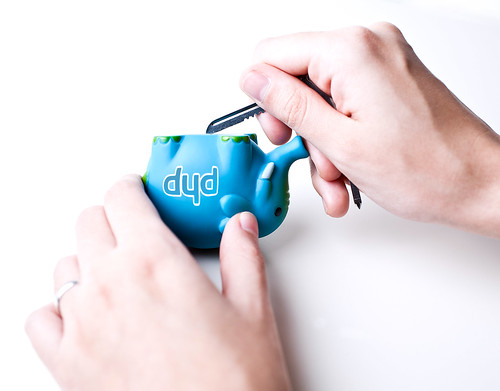
Last week I introduced you to the elePHPant, and showed you how to differentiate the PHP in your code from everything else. But that doesn’t actually tell you what’s happening between the PHP tags… which is kind of the entire point of learning about PHP, right? So today we begin to dissect the elePHPant, and learn about everything that goes on inside.
Functions
So how do you make your PHP “do stuff” to your webpage? Well, there are several ways, all of which you are likely to see in your WordPress theme (or in any PHP code), and all of which we will discuss in the next couple of weeks. It essentially comes down to two methods: you can put your logic (yes, you have to use logic; no, it’s not as difficult as it sounds) inline in the code for your webpage, or you can put it elsewhere and import it into your webpage using a PHP function.
It’s similar to what we said about using class attributes to apply the same style multiple places in your code, or using a separate file for your header so that you can import exactly the same header into every page without having to repeat the code. If you’re only going to use a certain block of PHP code once in your entire website, then it makes perfect sense to put it inline. However if you plan to use the same code many times in your website, it’s far better to put it in a separate function (usually in a separate file such as functions.php), and then just call that code using the name of the function.
Okay, so how do you know if something is a function, and not… something else? That’s easy. Your basic PHP function looks like this:
<?php myFunction(); ?>
This breaks down into three sections:
- Function Name: We called this function, “myFunction”. Notice that there are no spaces in the function name – instead we use something called camelCase, where the first letter in every word is capitalized. You are generally recommended to use only upper- and lower-case letters in your function names. Numbers are allowed but discouraged, and function naming conventions say you should only use underscores under very specific circumstances.
(However, many people use underscores where you might find spaces, instead of camelCase, like this: “my_function”. If you’re working as a professional programmer, you have to use the method that everyone else in your company uses. However if you’re just coding for yourself, you can really use whichever you’d like… so long as you never. ever. use spaces in your function names.)
Function names should always begin with lower-case letters, and they should describe what the function does – you wouldn’t want to name your function “header” (what does it do to the header?), but instead something like “getHeader” (this tells you the function gets the header). - Parentheses: You always must have the parentheses directly after the function name. When you have parameters to pass into the function, said parameters go between the parentheses. (What are parameters? Bear with me a moment more, and I’ll explain.) But even if you have no parameters, the parentheses must be there. Always.
- Semi-Colon: Every expression in your code must end with a semi-colon. It’s kind of like the period at the end of a sentence – it signifies that you’re at the end of the expression.
Easy peasy, right?
Parameters
Sometimes you want your function just to give you something – like the getHeader();
function, which will always give you the exact same header every time you call it. This is normal, and a perfectly valid function. But what if you want to take something in, do something to it, and spit something else out? For example, (this is a super simplified example, bear with me) what if you wanted to take in a number, multiply it by 7, and then return the result?
That’s what parameters are for.
The function would look a little something like this:
<?php function multiplyBySeven($int) { $int = $int * 7; return $int; } ?>
So when you call the function, if you did this: multiplyBySeven(4);
you would get back the number 28, or if you did this: multiplyBySeven(12);
you would get back the number 84.
Those things between the parentheses are called parameters. A function does not have to take in any parameters, or it can take in many parameters, or it can even take in optional parameters. For example, you usually wouldn’t pass in any parameters into the getHeader()
function, however if you look at the documentation for it, you’ll see that if you wanted to (and had reason to), you could pass in an optional parameter. Or take a look at our multiplyBySeven($int)
function – that takes in one parameter.
So how do you know what parameters are supposed to be passed into a function? This is where the internet comes in handy, because there are far too many existing functions for me to explain all of them. If you’re wondering about a function defined by the default WordPress theme, all you have to do is search for WordPress
and the function name. That should return a link to a page in the WordPress Codex, which is where the documentation for all the WordPress functions (and much more) exists. If it’s not there? Do a search for PHP
and the function name, and you’ll likely get a link to a page within the PHP Manual. This is where much of the more general PHP documentation is. And if you still can’t find it? There’s a good chance it’s a custom function… so check in the files within your theme, like functions.php
.
I’d Tell You More, But…
… goodness geeze, I’m long-winded. I was all ready to move on and explain why all those dollar signs are there in your code, but then I looked at everything I’ve already tried to stuff in your brain today and I realized I didn’t want to make anyone’s head explode.
But we’ve just barely broken the skin in our dissection of the infamous elePHPant so come back next week, when we talk variables and I have an itsy bitsy confession to make.
And if you ever have any coding questions, please don’t hesitate to ask! You can always leave a comment or hit me up on twitter… hey, maybe your question will even get its own blog post!
4 Comments
Pingback: PHP: Variables – SushiCodes
Pingback: PHP: On One Condition… – SushiCodes
Pingback: PHP: Wrapping Things Up – SushiCodes
Pingback: HTML/PHP: Sidebars, and What Have YOU Done Lately? - SushiCodes