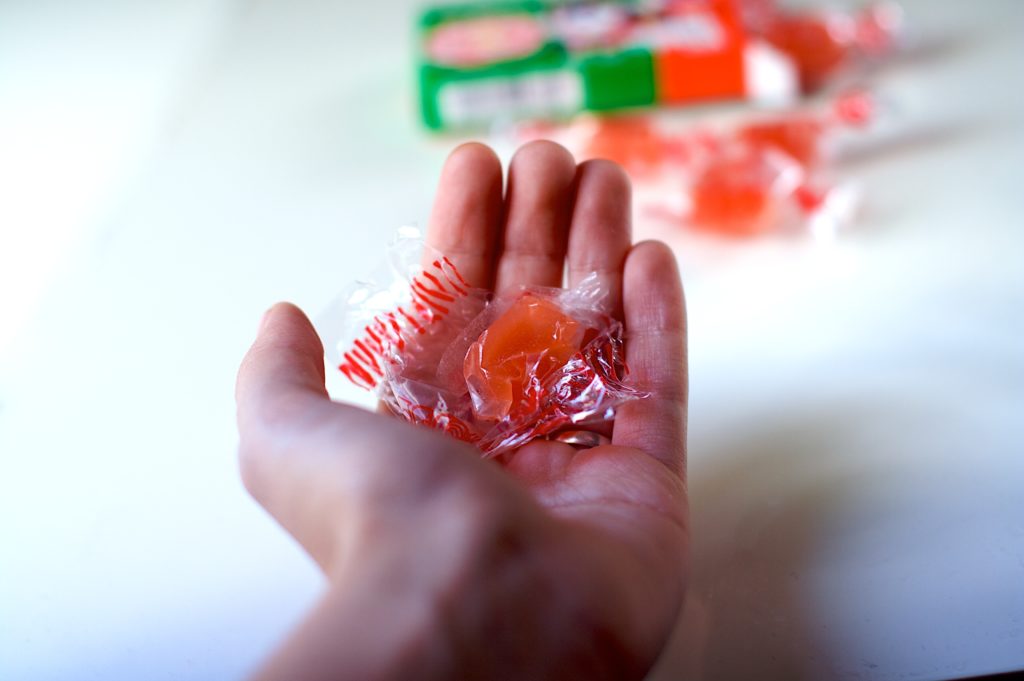
For the last couple of weeks, we’ve talked about functions and variables in your PHP code. But that’s not nearly all that there is to PHP. After all, there’s more to functions than just passing variables around (we have to do something to these variables, else what’s the point?) and functions aren’t the only way you can insert PHP functionality into your website.
It Must Meet These Conditions…
“If the user is logged in, they’re allowed to edit their profile. But if they’re not logged in, we’d better not let them even think about editing profiles.”
That sound familiar? It should. There are all sorts of websites out there that do something like this: Facebook, Twitter, Gmail… even your own WordPress sites. After all, you don’t want some stranger to be able to come in and make changes to your website without being logged in, right?
So how would you tell your code to do this? Just use a simple if/else statement. To put it in plain English, it’s something like this:
if (user logged in) { let them make changes } else { tell them "Sorry, you have to be logged in to do that." }
(FYI, this format is something known as “pseudo-code”. Some programmers will write their code in plain English like this first, so they know exactly what needs to be done before they start coding. Then they translate it into actual code.) Although real user management systems are a bit more complicated, it really all boils down to a basic if/else statement.
Let’s break it down. First, you have the word “if”, and a condition between the parentheses. If this condition is true (if the user is logged in), then this will tell your browser that it should execute the code between that first pair of brackets (let them make changes). If it isn’t true, then it won’t execute that code and it’ll move on to the “else” statement, and execute the code between those brackets (tell them “Sorry, you have to be logged in to do that.”). Make sense?
But what happens if you need more than a simple true/false condition? What if, say, you want to display something different depending on what day of the week it is? Well then, you could do something like this:
if (Monday) { print "Drag yourself out of bed, it's Monday." } else if (Tuesday) { print "The week's still getting started, it's Tuesday..." } else if (Wednesday) { print "Today's hump day..." } else if (Thursday) { print "It's Thursday! The Big Bang Theory is on today!" } else if (Friday) { print "TGIF!" } else if (Saturday or Sunday) { print "It's the weekend!" } else { print "Something went wrong... I'm pretty sure that's not a day of the week." }
You see that the code will move through each “if” and “else if” statement until it finds one that’s true. Today is Thursday, so it will skip over the first three statements because they will all be false, and only execute the Thursday code and print “It’s Thursday! The Big Bang Theory is on today!” If all the “if” statements are false, then it will end up at that last “else” statement.
(Yes, this could and probably should all be done using a switch statement, BUT we’re discussing if statements today, so we’ll get there when we get there!)
Taking Off the Training Wheels
As you can see, I wrote that entire if/else statement in pseudo-code. What would it look like if I wrote it in real code?
if (strcmp(date("l"), "Monday") == 0) { echo "Drag yourself out of bed, it's Monday."; } else if (strcmp(date("l"), "Tuesday") == 0) { echo "The week's still getting started, it's Tuesday..."; } else if (strcmp(date("l"), "Wednesday") == 0) { echo "Today's hump day..."; } else if (strcmp(date("l"), "Thursday") == 0) { echo "It's Thursday! The Big Bang Theory is on today!"; } else if (strcmp(date("l"), "Friday") == 0) { echo "TGIF!"; } else if (strcmp(date("l"), "Saturday") == 0 || strcmp(date("l"), "Sunday") == 0) { echo "It's the weekend!"; } else { echo "Something went wrong... I'm pretty sure that's not a day of the week."; }
It’s not as complicated as it looks. Those “if” and “else” parts are exactly like they were in our pseudo-code, so that’s nothing difficult. And we talked about how to use “echo” in your code last week, remember? (Don’t forget the semi-colons after each line of your code within the “if” statements!) The only thing that’s really new is that scary-looking stuff between the parentheses. Your conditions.
If you look closely, you’ll see that it’s just a couple of functions. You can tell because they’re of the form name(parameters)
.
“But there’s no semi-colon!” That’s okay. When you’re putting stuff between parentheses, like parameters in your functions or conditions in your “if” statements, you almost never use semi-colons. Except with “for” statements, but we’ll get to that later.
The first function I used was date($format)
. I knew I needed a function that would tell me what day of the week it is. The date function does exactly that. The parameter you pass in tells the function what information it should return about today’s date. I passed in “l” (lowercase L), which tells it that I want to know what day of the week it is. If instead I wanted it to tell me that today is Thursday, February 18, 2010 then I would pass in “l, F j, Y”. How do I know what letter(s) to use? I look at the PHP documentation for the date function, which explains all the parameters that I can pass into it.
Then I used strcmp($string1, $string2)
. “strcmp” stands for “string compare” – all it does is compare two strings to see if they are the same. In my if/else statement, I need to know which day of the week the date function returned so I know which condition will be true.
To figure that out, I use the strcmp function to compare the value that the date function returned to each day. If the values of the two strings are the same, strcmp
will return 0. So for the first condition, we compare the day to “Monday”. Since today isn’t Monday, it won’t return 0. And since it doesn’t return 0, then strcmp() == 0
won’t be true, so it will move on to the next function. But when it gets to “Thursday”, strcmp
will return 0 because today is Thursday. And since 0 == 0
, the condition is true! So it will print out “It’s Thursday! The Big Bang Theory is on today!” (What? Yes, I’m a nerd. But you knew that already.)
Why do we use two equal signs? If you want to set a variable equal to something, you use one equal sign. If you want to see if a variable is equal to something, you use two. So for these conditional statements, where we want to see if our strcmp
function is equal to zero, we use two equal signs (I’ll explain this more in a few weeks when we talk about PHP operators).
If you’re looking at the code, there might be one more thing that looks unfamiliar. What is that ||
thing? In our code, we want to print out “It’s the weekend!” if it’s the weekend of course, which means Saturday OR Sunday. So we need a way to say that the condition is true if today is Saturday OR if today is Sunday. That’s what the ||
operator does: it says, our condition will be true if strcmp(date("l"), "Saturday") == 0
or if strcmp(date("l"), "Sunday") == 0
. Easy! (There are other operators, like AND or NOT, that we’ll discuss in future posts.)
Going in Circles…
Next week, we’re getting a little loopy. ‘While’ loops, ‘for’ loops, ‘foreach’ loops… we’re going to be going around and around in circles.
But don’t worry. I’ll try not to make you too dizzy.
2 Comments
Pingback: PHP: Getting Loopy – SushiCodes
Pingback: PHP: For, While, and other Loopy Things – SushiCodes