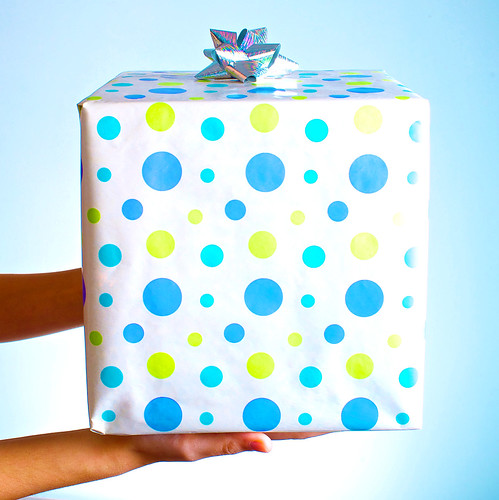
Last week we talked about what functions are in PHP, and I promised that this week I would explain more about what goes into a function and why on earth there are so many dollar signs in PHP code. So to put things into context before we jump right in, remember that we were discussing functions, kind of like this:
function multiplyBySeven($int) { $seven = 7; $int = $int * $seven; return $int; }
multiplyBySeven
is the name of our function (which, as you might guess, multiplies a number by 7), and we pass a parameter $int
into it.
These Things Called Variables
“Alrighty then, Allison, tell us… what are all those dollar signs about? I thought we were talking about coding, not economics.”
Those dollar signs mean we’re talking about variables. Variables are basically containers that hold values that can change or vary throughout your code (hence the name).
It’s kind of like a present. Say you put something in a box and give it to a friend. Maybe that friend doesn’t change anything, and (*gasp*) re-gifts the present to someone else exactly as they got it. (You can pass the variable around into other functions or use it to change another variable without changing itself in any way.) Or maybe they just reuse the container, and put in another gift (a different value) before passing it along to someone else. (You can alter the variable’s value or even replace it with another value before you pass it elsewhere.) Make sense?
Another good way to explain how variables work is to show an example: Just as in our parameters example last week, you see that the variable $num
starts out with a value of 10. But by the time it is returned, it has a value of 50. The ability to alter or change the value of variables any time you need to makes them very valuable in programming languages.
The naming conventions are essentially the same as for functions, except that variable names in PHP must always start with a dollar sign.
“But… $int
has a dollar sign. I thought it was a parameter, because it’s between those parentheses after the function name?”
It is. It’s also a variable. You see, when you pass a value into a function, you want to be able to use that value within the function. Otherwise, what’s the point of passing it in? And in order to move a value around within a function, you need to assign it to a variable first. Therefore, anytime you pass a parameter into a function, it will always be assigned to a variable. But not all variables are parameters (like $seven
in that first example). Get it?
Although in all our examples so far we’ve assigned integers to our variables, there are lots of other value types that can be assigned to variables as well. For example, you can have boolean variables ($boolTrue = TRUE; $boolFalse = FALSE;
), string variables ($stringVar = "sushi";
), float variables ($float = 14.75;
) (recall that integers are whole numbers, like 1 or -7. Floats, also known as floating-point or ‘double’ numbers, are non-integers that have decimal points), or even arrays and objects (which are way outside the scope of almost any WordPress theme, so I won’t go into them here.) You can even have a variable point to nothing at all ($nullVar = NULL;
).
But what if you don’t want your variable to ever change? You use a constant. These are relatively rare in WordPress themes–you’re far more likely to see a variable than a constant. But just so you know, constants are always in all caps, and instead of defining them like you do variables ($num = 7;
), you have to use the define()
function to instantiate them.
That’s more than you need to know for WordPress themes, but if anyone’s curious, let me know in the comments and I’ll gladly explain further.
Little White Lie
Last week, remember how I told you that your PHP code always, always has to be surrounded by the <?php and ?> tags? Well, that isn’t entirely true. I mean, it is, I wasn’t lying to you, but… well… *sigh*
Technically, there are a few other PHP tag styles that you could use, such as <% %>
, or <? ?>
. However, it’s not recommended. These styles are much more rare than the <?php ?>
tags, and not nearly as widely supported. So really no reason to talk about it, right?
The reason I bring it up is that I have seen some themes use PHP tags that are generally not recommended, and I figure you should know what’s going on even if it’s not the best way of doing things. They look something like this:
<?=get_bloginfo('stylesheet_directory')?>
What that does is put the value that you get from that function directly into your code. (It essentially says, “What goes right here is equal to this function.”) I believe it’s supported by almost all browsers, but technically it’s not recommended. Instead, you’re supposed to do something more like this, which does exactly the same thing:
<?php echo get_bloginfo('stylesheet_directory'); ?>
(That ‘echo’ before the function merely means that it’s supposed to “echo” the value from the function… or print it out into your code, so it’s visible in the final webpage.)
Moving On…
Thank goodness we got that all straightened out. We know what variables are now, but does this mean we’re finished with our PHP lesson?
Nope. Not even close.
Next,we discuss conditionals. Conditional statements, that is. Not the “If I told you, I’d have to kill you” types, but more like “If a user is logged in, then they get to see this page,” or “If you don’t read next week’s post, then you will be destroyed by the secret internet monster.”
If you think I’m joking, then you better come back next week to find out, huh?
1 Comment
Pingback: PHP: On One Condition… – SushiCodes