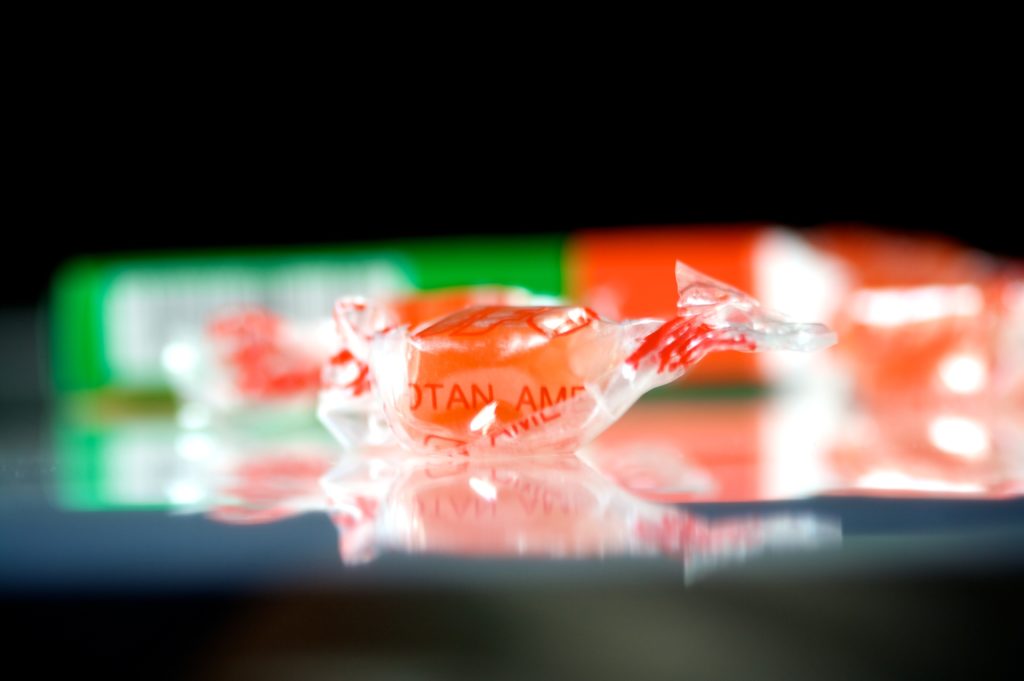
If you’ve been reading my posts about coding the last few weeks, then you’re pretty much ready to deal with any of the PHP that you come across in a WordPress theme. Heck, maybe you’re even ready to write your own PHP! I’m so proud… you’re all such good students.
But before we move on to the next part of your theme – the actual HTML in the body that displays what you see on your website – there are a few last things to wrap up regarding PHP.
Operators
There’s one last thing that we haven’t discussed much in PHP, that you’re guaranteed to see in almost any PHP code out there. Operators. It might seem like we’re taking a step back into middle school math, but this will be painless. I promise.
First, we have the arithmetic operators: +
, -
, /
, *
, %
. These do exactly what you’d expect: add, subtract, divide, multiply, and take the modulus (the remainder of one number divided by another. 5 % 2 is 1). You can also negate a value by putting -
in front of it. Easy.
Then, there are the assignment operators. =
is obvious, you’re just setting one value equal to another, like this: $a = 5
. Then there’s +=
which means that you add the value to the variable. So $a += 2
would be 7, because we already set $a
to 5. That works for numbers, but what about strings? Here you use .=
which tells you to concatenate one string onto the end of other. Pretty much just sticking them together. So if we started with $b = 'Hello ';
and then did $b .= 'world!';
, $b
would end up as 'Hello world!'
. You do something similar if you want to stick a variable in the middle of a string. You could just do $c = $b . " What's up?";
and it would show up as Hello world! What's up?"
(Notice that I used double quotes to surround the string there. Since we had an apostrophe in “what’s”, I didn’t want it to be read as a closing single quote, which would screw things up. Luckily, in PHP you can just use double quotes instead, and avoid that problem.)
Next are the comparison operators. We already know that =
sets a variable equal to a value. But what if we want to test to see if two things are equal? We definitely don’t want to set the variable equal to the value we’re testing for. That would be bad. So instead we use ==
or ===
. $a == $b
will be true if $a
is equal to $b
. $a === $b
is the same, but also adds the constraint that $a
and $b
have to be the same type. (But why? Well, what if you wanted to test to see if $a
is equal to the boolean value true
? If $a
is any non-zero, non-null value, then when you translate it to boolean it shows up as true
. But that’s not what we want. So instead we’d use ===
to say that it’s only true if $a
is the boolean value true
, and if it’s not boolean, then it’ll show up as false
.) Then we have !=
which tests to see if two values are not equal, and !==
to see if two values are either not equal or not of the same type. The opposite of ==
and ===
, if you will. The other four comparison operators should be familiar from your old math classes: <
, >
, <=
, >=
simply test to see if one value is less than, greater than, less than or equal to, or greater than or equal to another.
Then there are the incrementing and decrementing operators. ++
increments (adds 1 to) a variable. --
decrements (subtracts 1 from) a variable. Put it beforehand if you want to increment/decrement before the value is returned (if you start with $a = 1
, echo ++$a;
will print out ‘2’). Put it afterward if you want to increment/decrement after the value is returned (if you start with $a = 1
, echo $a++;
will print out ‘1’, and then if you echo $a;
after that, it will print out ‘2’).
Next we have logical operators. We’ve already talked about &&
and ||
… ($a && $b)
means that both $a
and $b
have to be true in order for the entire expression to be true, and ($a || $b)
means that either $a
or $b
or both have to be true for the expression to be true. (You can also use and
instead of &&
, and or
instead of ||
.) If you want either $a
or $b
to have to be true, but NOT both to have to be true, then you can use $a xor $b
. Lastly we have !
, which means not. (!$a)
means that $a
has to be false for the expression to be true. Make sense?
Teacher, Teacher, I Have A Question
Now that we’ve explained the basis of PHP for you, where can you go to find more information?
Well, you can always come back here, of course. For now I’m moving on to talk about HTML, CSS, and JavaScript, but you can be sure there will be more posts about PHP in the future as well.
PHP.net is an excellent resource. Any time you ever have to look up a function that you (or your web developer) didn’t create on your own, chances are you’ll find the documentation for it here. And it goes the other way around too – whenever you need to figure out how to do something (for example, what if you want to display today’s date? or change a string to all lowercase?) you can do a search for it, and 90% of the time you’ll find a pre-made function that does exactly what you need, on PHP.net. And while you’re there, check out the comments on every page – there you’ll find discussion and examples about how other programmers have used these functions.
w3schools.com is another great resource. While PHP.net is all about documentation, w3schools.com tends more towards tutorials and examples how to use things.
If you want to purchase a book about PHP, Head First PHP & MySQL is an excellent resource. I love the Head First series for learning any sort of programming language – they explain things in ways that are very easy to understand, and even have games at the end of every chapter to help you remember what you learned. I have a couple of the Head First books from back when I was first learning, and I can definitely vouch for how helpful and easy to use they are.
I Am Here For You!
If there’s anything else – anything at all – you want to know about PHP, or coding in general, just ask! Even if it’s something that can be found in any of those references, I’m always happy to help. Whether it’s something confusing from the code in your theme, or questions about different web technologies, or anything else coding- or tech-related, I’m here for you. Really. I want to help you guys. And maybe you’ll even get a blog post written specifically to answer your question!